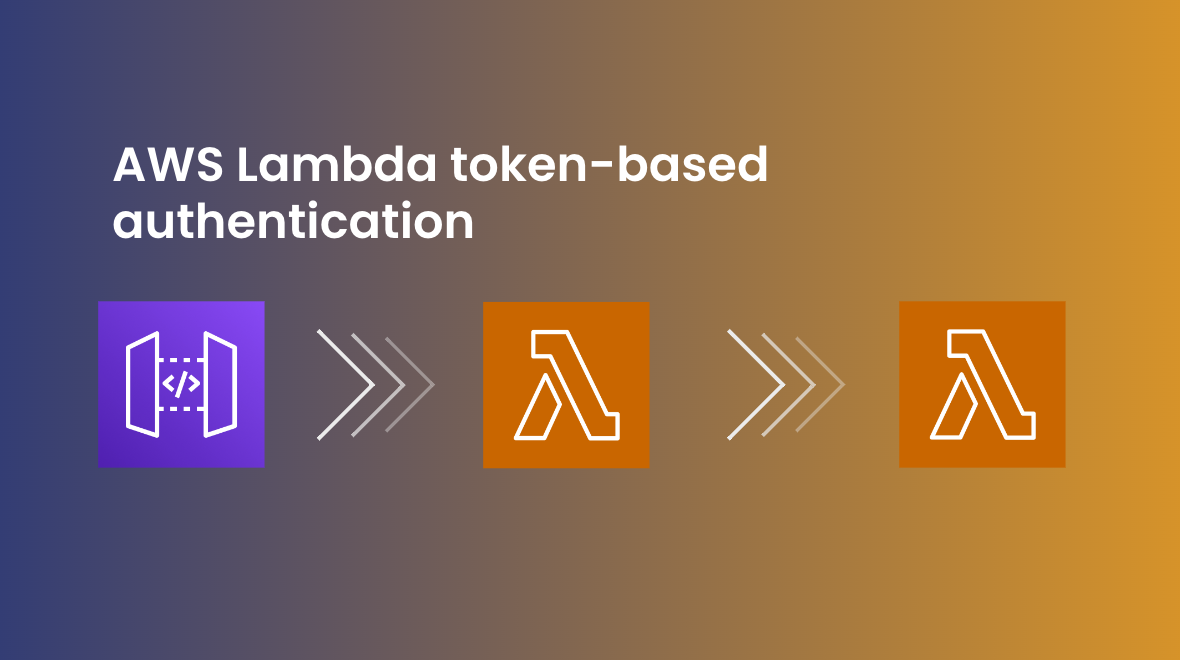
Setting Up AWS Lambda with Bearer Token Authentication using AWS API Gateway
Gaël Grosch
/
AWS Lambda makes it very easy to deploy almost any type of code including python code. If you have low (as it might be when starting out) or bursty usage, it offers a great and cost-efficient solution to quickly develop new solutions.
Of course, unless you are developing a public API, you will need to secure your API. A common method for this is using the AWS API Gateway which can be configured to use a Lambda function to authenticate the user with a “Lambda authorizer”.
There are two types of Lambda authorizers:
-
A token-based Lambda authorizer such as a bearer token
-
A request parameter-based Lambda authorizer
In this article, we will look into how to setup the first option: a token-based Lambda authorizer.
What You’ll Need
- AWS account
- Basic understanding of AWS services
- An existing AWS Lambda that you want to protect
- An existing AWS API Gateway
Step 1: Setting Up Your AWS Lambda Authorizer
To get started:
- Navigate to AWS Lambda.
- Create a new Lambda function. Choose ‘Author from scratch’, and select Node as your runtime.
Use the following code. Please change the secret token to your own secret token:
export const handler = async (event) => {
const token =
event.headers && event.headers.authorization
? event.headers.authorization.replace("Bearer ", "")
: "";
if (token === "YOUR_SECRET_TOKEN") {
return {
isAuthorized: true,
context: {
// Put additional content here if desired
},
};
} else {
return {
isAuthorized: false,
context: {
// Put additional content here if desired
},
};
}
return response;
};
And create a test event with:
{
"header": {
"Authorization": "Bearer YOUR_SECRET_TOKEN",
"Content-Type": "application/json"
}
}
If the test is successful, you should see the following output:
{
"isAuthorized": true,
"context": {}
}
That means that the Lambda function is working as expected.
Step 2: Configuring AWS API Gateway with Lambda Authorizer
We will configure the following setup where the Lambda authorizer is used to protect the Lambda function:

Here are the steps:
- Navigate to AWS API Gateway.
- Select your API (HTTP).
- Go to ‘Authorizers’ and select “Create authorizer”
Configure it with the following settings:
Authorizer Type = Lambda
Lambda Function = {Select the Lambda function you created in step 1}
And leave the rest as default:
Lambda Event Payload = Token
Payload Format Version = 2.0
Response Mode = Simple
Identity Sources = "$request.header.Authorization"
Step 3: Test and enjoy
That’s it, you have now set up a token-based Lambda authorizer for your AWS Gateway. You should be able to test accessing your protected endpoint in Postman or with a curl request:
curl --location 'https://{your_lambda_link}.amazonaws.com/{your_endpoint}' \
--header 'Authorization: Bearer secretToken' \
--data ''